Send Incident into Master Flow
Overview
This guide will walk you through the steps to create and test an example of a trigger which will essentially send new incidents into the DG Master Flow
Prerequisites
• Access to Oracle Service Cloud with admin privileges*.
• Access the Oracle Service Cloud platform from a Windows machine
Steps to install Oracle Service Cloud platform on a Windows machine:
- Access the launch page for Oracle (this has a similar structure to the following URL: [/cgi-bin//php/admin/launch.php](/cgi-bin//php/admin/launch.php))
- Click on Install Oracle Service Cloud
- Once finished, login with your credentials
*To add a custom script (CP), you will need to have access to the Process Designer.
Steps to create the DG Send Incident into Master Flow trigger on Oracle
To create a trigger in Oracle which sends incidents into the DG dashboard, you need to first create an Object Even Handler and then add this object event handler to the incidents.
Adding DG credentials in the Configuration Settings
Before adding the CP into Oracle, we will save the DG sensitive information in the Configuration Settings. This means that the DG Master Flow ID, DG API Key and DG API Secret are not exposed.
To add new records into Oracle, follow the next steps:
-
Go to Configuration --> Site Configuration --> Configuration Settings:
-
At the top left, click on New and Text
-
Add a the name to the key (CUSTOM_CFG_DG_API_KEY, CUSTOM_CFG_DG_API_SECRET and CUSTOM_CFG_DG_FLOW_ID)
-
Click on Save and make sure this new custom record is being added into the site configurations
-
Repeat steps 2, 3 and 4 for all the records (CUSTOM_CFG_DG_API_KEY, CUSTOM_CFG_DG_API_SECRET and CUSTOM_CFG_DG_FLOW_ID)
Adding the Object Event Handler in Oracle
An Object Event Handler (OEH) is a is a piece of custom code that responds to specific events related to objects in the system. In Oracle, these events can include the creation, update, or deletion of records such as incidents, contacts, or custom objects. OEHs allow you to implement custom business logic that is triggered automatically when these events occur.
The script we are implementing into the system does the following:
- Fetches system configurations.
- Sends incident details (ID and Subject) to a specified external DG API.
- Logs responses and errors for debugging purposes.
Once the system parameters are setup in the system, you can add the CP:
-
Go to Configuration --> Site Configuration --> Process Designer:
-
Click on New
-
This will open the UI for adding a CP as an OEH. Before doing anything, make sure that the box Execute Asynchronous is ticked
-
Add a name (preferably DGIncidentHandler_Master)
-
Leave the PHP version as it is (the CP below is currently working with PHP v5.6, if you experience any issues, please contact DG support)
-
Upload the following CP:
<?php
/*
* CPMObjectEventHandler: DGIncidentHandler
* Package: OracleServiceCloud
* Objects: Incident
* Actions: Create
* Version: 1.3
*/
use \RightNow\Connect\v1_3 as RNCPHP;
use \RightNow\CPM\v1 as RNCPM;
class DGIncidentHandler implements RNCPM\ObjectEventHandler
{
public static $isProd = null;
// main function which is the entry point
public static function apply($run_mode, $action, $incident, $n_cycles){
self::$isProd = 1;
return self::sendToDG($incident);
}
public static function sendToDG($incident)
{
$url = "https://flow-server.eu.dgdeepai.com/v2/execution";
$username = RNCPHP\Configuration::fetch('CUSTOM_CFG_DG_API_KEY')->Value;
$password = RNCPHP\Configuration::fetch('CUSTOM_CFG_DG_API_SECRET')->Value;
$flow_id = RNCPHP\Configuration::fetch('CUSTOM_CFG_DG_FLOW_ID')->Value;
$incident_id = $incident->ID;
$subject = $incident->Subject;
$incident_data = $incident;
$data = [
'action_id' => strval($flow_id),
'external_id' => strval($incident_id),
'block_for_flow_result' => true,
'inputs' => [
'Subject' => $subject,
'Id' => $incident_id,
]
];
$auth = base64_encode("$username:$password");
load_curl();
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Accept: application/json',
'Content-Type: application/json',
'Connection: Keep-Alive',
"Authorization: Basic $auth"
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_TIMEOUT, 5);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, TRUE);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
$response = curl_exec($ch);
if (curl_errno($ch)) {
$error_msg = curl_error($ch);
self::mlog("error from DG API", $error_msg);
return $error_msg;
}
if ($response = json_decode($response)) {
self::mlog("response from DG API", $response);
return $response;
}
}
public static function mlog($msg, $data)
{
if (!DGIncidentHandler::$isProd) {
$date = date('Y-m-d H:i:s', time());
$logMsg = "<br>$date<br>$msg<br>";
print_r($logMsg);
print_r($data);
}
}
}
class DGIncidentHandler_TestHarness implements RNCPM\ObjectEventHandler_TestHarness
{
public static function setup()
{
}
public static function fetchObject($action, $object_type)
{
$incident = $object_type::first('ID > 0');
return $incident;
}
public static function validate($action, $object)
{
return true;
}
public static function cleanup()
{
return;
}
}
Note that in the above CP, the site configuration parameters were named as CUSTOM_CFG_DG_API_KEY, CUSTOM_CFG_DG_API_SECRET and CUSTOM_CFG_DG_FLOW_ID. If you have used another name, please amend this.
Once this is added, click on Save and after it is saved, click on Test. This CP already contains a testing harness (DGIncidentHandler_TestHarness) which provides methods to set up, fetch objects, validate, and clean up for testing purposes. After testing, if successful, you should see an empty pop up message coming up. This means no errors were found when running this test.
Go to your Master Flow in the DG Dashboard and check if there have been any executions. If so, this means that the trigger is working correctly.
Adding the new OEH into the incidents
Once the OEH is created, you will need to add this new logic into your incidents. For this, within the same page (Process Designer), expand the menu OracleServiceCloud, click on Incident and add the newly created OEH for all the incident interfaces under Create.
Note that the checkbox Can Suppress needs to be disabled / unticked.
Once this is done, click on Deploy which will push all the changes live. After it is deployed, monitor your Master Flow to ensure you are getting all new incidents.
Troubleshooting
Invalid ID: No Configuration record found with LookupName = ...
The following error message indicates that one of the records saved in the site configuration is not found:
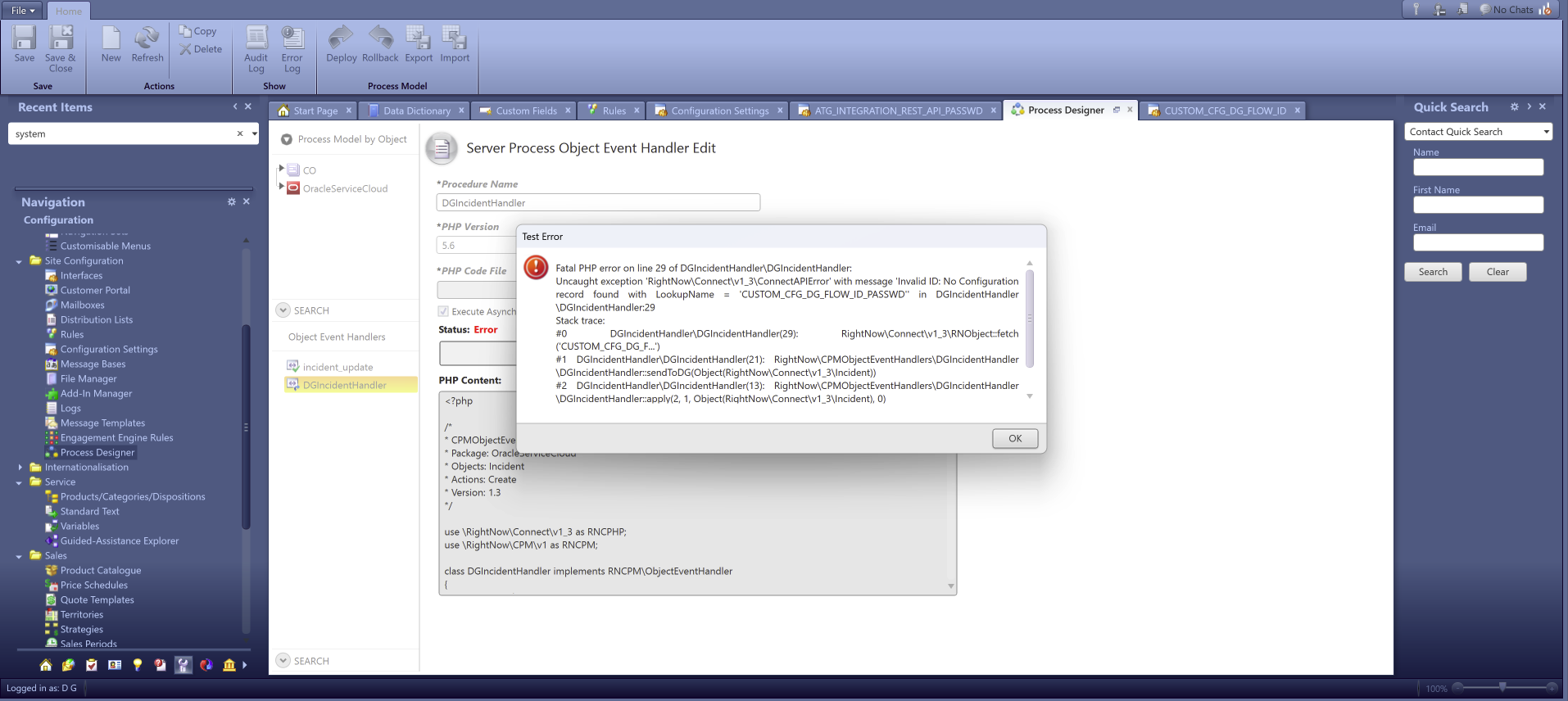
This can be due to multiple issues:
- Check that the key name is exactly the same as in the CP
- Ensure that the record exists and it didn't show any errors when trying to save it
- If the issue persists, try to test the CP by using hardcoded variables and not the ones from the site configuration
Updated 9 months ago